The 20 Most Common Use Cases for JavaScript Arrays
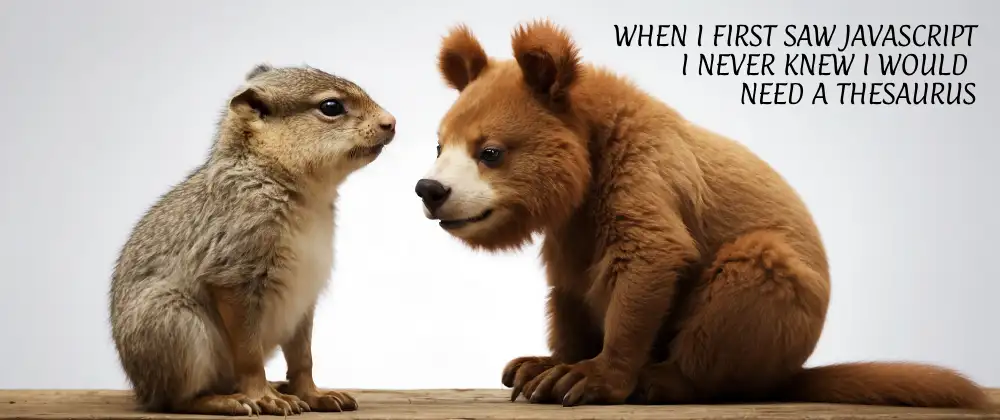
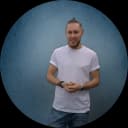
URL copied to clipboard
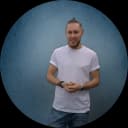
Splice, slice, pop and shift. Is the array sort method stable and not in-place? It's not easy to remember all JavaScript array methods and what the difference between them are. They are all synonyms, as if they where taken directly from a thesaurus.
This array cheat sheet lists all the array methods you usually need in JavaScript, and the ones you don't need, is not here. Simple as that! To make it even easier for you, I have based the cheat sheet on common use cases.
New challenge, try to list all array methods without forgetting one of them
Immutability
Before we begin, a notice about immutability. All functions used in this article are immutable, which shortly means, that you never mutate your original array when you use these methods. Immutability is very important in modern JavaScript, at least according to this Stack Overflow guy (and the rest of the Internet).
Even though each of these methods is immutable on their own, you are not totally safe against immutability. If you include mutable data types (arrays, objects, functions) in your immutable arrays, you can still mutate the mutable data within the array. We call such arrays to be shallowly immutable, in contrast to deeply immutable arrays which only includes immutable items.
Array Use Cases
- Add Element to Start of Array
- Add Element to End of Array
- Remove an Element From Start of Array
- Remove an Element From End of Array
- Insert Element at Index in Array
- Replace an Element at Index in Array
- Remove an Element at Index in Array
- Remove an Element By Value From an Array
- Remove Objects By Property From an Array
- Check if Array Includes an Element
- Check if Array Includes Object with Property
- Check if All Objects in an Array has a Property
- Convert Array to an Object
- Convert Array of Objects to an Object
- Convert Object to an Array
- Combine Two Arrays
- Sort an Array
- Sort an Array of Objects
- Reverse an Array
- Remove Duplicates From an Array
1. Add Element to Start of Array
To prepend an item to the beginning of an array, use array spreading.
const arr = [1, 2, 3];
const result = [0, ...arr];
console.log(result);
// [0, 1, 2, 3]
Do not use the unshift method, that will mutate the original array.
2. Add Element to End of Array
To append an item to the end of an array, use array spreading.
const arr = [1, 2, 3];
const result = [...arr, 4];
console.log(result);
// [1, 2, 3, 4]
Do not use the push method, that will mutate the original array.
3. Remove an Element From Start of Array
To remove the first item in an array, use slice method.
const arr = [1, 2, 3];
// Keep index 1 and everything after that.
const result = arr.slice(1);
console.log(result);
// [2, 3]
Do not use the shift or splice methods, they will mutate the original array.
4. Remove an Element From End of Array
To remove the last item in an array, use slice method.
const arr = [1, 2, 3];
// Keep index 0 and everything after that, except one element at the array.
const result = arr.slice(0, -1);
console.log(result);
// [1, 2]
Do not use the pop or splice methods, they will mutate the original array.
5. Insert Element at Index in Array
To add an item at a specific index of an array, use the toSpliced method.
const arr = [1, 2, 3];
// Keep index 1, delete 0 elements, add the element "one point five" and keep everything after that.
const result = arr.toSpliced(1, 0, "one point five");
console.log(result);
// [1, "one point five", 2, 3]
Do not use the splice method, that will mutate the original array.
6. Replace an Element at Index in Array
To replace an item from some index in an array, use the toSpliced or with method.
const arr = [1, 2, 3];
// Using toSpliced.
// Keep index 1, delete 1 elements, add the element "two" and keep everything after that.
const result1 = arr.toSpliced(1, 1, "two");
console.log(result1);
// [1, "two", 3]
// Using with.
// Copy the old array arr with index 1 replaced with "two".
const result2 = arr.with(1, "two");
console.log(result2);
// [1, "two", 3]
Do not use the splice method, that will mutate the original array.
7. Remove an Element at Index in Array
To remove an item from an array, use the toSpliced method.
const arr = [1, 2, 3];
// At index 1, delete 1 elements.
const result = arr.toSpliced(1, 1);
console.log(result);
// [1, 3]
Do not use the splice method, that will mutate the original array.
8. Remove an Element By Value From an Array
To remove a specific value from an array, use the filter method.
const arr = [1, 2, 3];
const result = arr.filter((element) => element !== 2);
console.log(result);
// [1, 3]
Do not use the indexOf together with splice method, that will mutate the original array.
9. Remove Objects By Property From an Array
To remove an object with a specific attribute from an array, use the filter method.
const arr = [{ num: 1 }, { num: 2 }, { num: 3 }];
const result = arr.filter((obj) => obj.num !== 2);
console.log(result);
// [{ num: 1 }, { num: 3 }]
Do not use the findIndex together with splice method, that will mutate the original array.
10. Check if Array Includes an Element
To check if an array contains a value, use includes method.
const arr = [1, 2, 3];
const result = arr.includes(2);
console.log(result);
// true
11. Check if Array Includes Object with Property
To check if an array contains an object with a property, use some method.
const arr = [{ num: 1 }, { num: 2 }, { num: 3 }];
const result = arr.some((obj) => obj.num === 2);
console.log(result);
// true
12. Check if All Objects in an Array has a Property
To check if every object in an array has a property, use every method.
const arr1 = [{ num: 1 }, { num: 2 }, { num: 3 }];
const result1 = arr1.every((obj) => obj.num === 2);
console.log(result1);
// false
const arr2 = [{ num: 2 }, { num: 2 }, { num: 2 }];
const result2 = arr2.every((obj) => obj.num === 2);
console.log(result2);
// true
13. Convert Array to an Object
To convert an array to a custom object, use reduce method.
// A function which maps a key to a value.
const arr1 = [1, 2, 3];
const result1 = arr1.reduce((acc, cur, index) => {
acc[`attr${index}`] = cur;
return acc;
}, {});
console.log(result1);
// { attr0: 1, attr1: 2, attr2: 3 }
// A function which count occurrences could look like this.
const arr2 = ["a", "b", "c", "c"];
const result2 = arr2.reduce((acc, cur) => {
if (acc[cur]) {
acc[cur] += 1;
} else {
acc[cur] = 1;
}
return acc;
}, {});
console.log(result2);
// { a: 1, b: 1, c: 2 })
// A function which maps elements in array to boolean values can look like this.
// I.e. convert array to object keys.
const arr3 = ["a", "b", "c"];
const truthValues = ["b", "c"];
const result3 = arr3.reduce((acc, cur) => {
acc[cur] = truthValues.includes(cur);
return acc;
}, {});
console.log(result3);
// { a: false, b: true, c: true })
14. Convert Array of Objects to an Object
To convert an array of objects to an object, use Object.assign method and array spread syntax.
const arr = [{ attr1: 1 }, { attr2: 2 }, { attr3: 3 }];
const result = Object.assign({}, ...arr);
console.log(result);
// { attr1: 1, attr2: 2, attr3: 3 }
15. Convert Object to an Array
To create an array from an object, use Object.keys, Object.values, or Object.entries, potentially together with a map method.
const obj = { a: 1, b: 2, c: 3 };
// Array of keys.
const result1 = Object.keys(obj);
console.log(result1);
// ["a", "b", "c"]
// Array of values.
const result2 = Object.values(obj);
console.log(result2);
// [1, 2, 3]
// Array of key-value objects.
const result3 = Object.entries(obj).map(([key, value]) => ({ key, value }));
console.log(result3);
// [{ key: "a", value: 1 }, { key: "b", value: 2 }, { key: "c", value: 3 }]
In some cases, it is useful to chain some map and filter methods to modify and filter out values.
const obj = { a: 1, b: 2, c: 3 };
// Array of squared values greater than 3.
const result1 = Object.values(obj)
.map((value) => value * value)
.filter((value) => value > 3);
console.log(result1);
// [4, 9]
// Array of key-value objects which has a value greater than 1.
const result2 = Object.entries(obj)
.map(([key, value]) => ({ key, value }))
.filter((keyValueObj) => keyValueObj.value > 1);
console.log(result2);
// [{ key: "b", value: 2 }, { key: "c", value: 3 }]
16. Combine Two Arrays
To combine two JavaScript arrays, use concat method or the spread syntax for arrays.
const arr1 = [1, 2, 3];
const arr2 = [4, 5, 6];
// Concat method is faster.
const combinedArray1 = arr1.concat(arr2);
console.log(combinedArray1);
// [1, 2, 3, 4, 5, 6]
// Spread syntax may be more readable.
const combinedArray2 = [...arr1, ...arr2];
console.log(combinedArray2);
// [1, 2, 3, 4, 5, 6]
Do not use the push method, that will mutate the original array.
17. Sort an Array
If you want to sort an array by value, use toStorted method.
The toStorted method is stable, meaning that it keeps the order of elements which are equal to each other intact. The method is not in-place, which usually is a good thing, since it means that it won't mutate the existing array.
// To sort strings.
let arr1 = ["b", "c", "a"];
const result1 = arr1.toSorted();
console.log(result1);
// ["a", "b", "c"]
// Note: Numbers are sorted by their toString value,
// not by their numerical value!
const arr2 = [10, 1, 5];
const result2 = arr2.toSorted();
console.log(result2);
// [1, 10, 5]
// To sort numbers, use a comparator.
const arr3 = [10, 1, 5];
const result3 = arr3.toSorted((a, b) => a - b);
console.log(result3);
// [1, 5, 10]
Do not use the sort method, that will mutate the original array since it does in-place sorting.
18. Sort an Array of Objects
To sort an array by value, use toStorted method with a comparator. A comparator is a function which determines which of two values that should be sorted first.
The toStorted method is stable, meaning that it keeps the order of elements which are equal to each other intact. The method is not in-place, which usually is a good thing, since it means that it won't mutate the existing array.
const arr = [{ num: 3 }, { num: 1 }, { num: 2 }];
// ObjA will be sorted before objB if comparator returns a positive value.
const byNumberAttribute = (objA, objB) => objA.num - objB.num;
const result1 = arr.toSorted(byNumberAttribute);
console.log(result1);
// [{ num: 1 }, { num: 2 }, { num: 3 }]
// More generic comparator.
const byAttribute = (attr) => (objA, objB) => objA[attr] - objB[attr];
const result2 = arr.toSorted(byAttribute("num"));
console.log(result2);
// [{ num: 1 }, { num: 2 }, { num: 3 }]
// Note. The comparator function must return an integer value.
// If you need to sort other data types, return 1, 0 or -1.
const arr3 = [{ letter: "c" }, { letter: "a" }, { letter: "b" }];
const alphabetically = (objA, objB) => {
if (objA.letter < objB.letter) {
return -1;
}
if (objA.letter > objB.letter) {
return 1;
}
// objA === objB
return 0;
};
const result3 = arr3.toSorted(alphabetically);
console.log(result3);
// [{ letter: 'a' }, { letter: 'b' }, { letter: 'c' }]
Do not use the sort method, that will mutate the original array.
19. Reverse an Array
To reverse all values in an array, use toReversed method.
const arr = [1, 2, 3];
const result = arr.toReversed(2);
console.log(result);
// [3, 2, 1]
20. Remove Duplicates From an Array
To remove duplicated elements in an array, use filter method or a set.
const arr = [1, 2, 3, 2, 1];
// Using filter method.
const result1 = arr.filter((item, index) => arr.indexOf(item) === index);
console.log(result1);
// [1, 2, 3]
// Using a set.
const result2 = [...new Set(arr)];
console.log(result2);
// [1, 2, 3]